[Author Prev][Author Next][Thread Prev][Thread Next][Author Index][Thread Index]
[pygame] `pygame.draw.line(...)` `width`
- To: "pygame-users@xxxxxxxx" <pygame-users@xxxxxxxx>
- Subject: [pygame] `pygame.draw.line(...)` `width`
- From: Ian Mallett <ian@xxxxxxxxxxxxxx>
- Date: Sat, 28 Apr 2018 14:08:14 -0600
- Delivered-to: archiver@xxxxxxxx
- Delivered-to: pygame-users-outgoing@xxxxxxxx
- Delivered-to: pygame-users@xxxxxxxx
- Delivery-date: Sat, 28 Apr 2018 16:08:37 -0400
- Dkim-signature: v=1; a=rsa-sha256; c=relaxed/relaxed; d=gmail.com; s=20161025; h=mime-version:sender:from:date:message-id:subject:to; bh=kNAnsSWX1+8KsJrdpLl9yMMfkYEXhH3O+keqfTxmyBw=; b=deDlRO4jr1/sc8vmQo7mkQ6kUShDZOAcqc8oAi05iXUZ5oo+34E6ZaPSvyRcQK4byS CgEInvE4esmiaBcgRJtOwX2V8n8zsqJcTsXwPXjXhsqhI3QZUYzgCh9+rtF0LtVpLShU u4GMsZCBuAKkFHp+ZTrsu3Ezgb+bWMXIk7TDjMBpPu+X0Sy0VbSvKpnfE8onbTzxn2RL 603kdRMWj5lJeHhKiFD91PGR8FY4B0KLkWmBDlM2TLoDna0U7vlXx5APM6RZh9z8DE8b LrIfq5OV1r+4H5EGSksqzxcFrvgR8E9IcAJzsXjrWDduGU8MaLaE57xLtPdLGp1xUgoI sy2w==
- Reply-to: pygame-users@xxxxxxxx
- Sender: owner-pygame-users@xxxxxxxx
Hi,
I don't remember noticing this behavior before, but it seems that the lines' widths are chosen so that the ends, which are also axis-aligned, are the given width. Thus, the lines can be up to about 29% thinner than one would expect.
Here's an example image; note esp. how the diagonal line appears too thin:
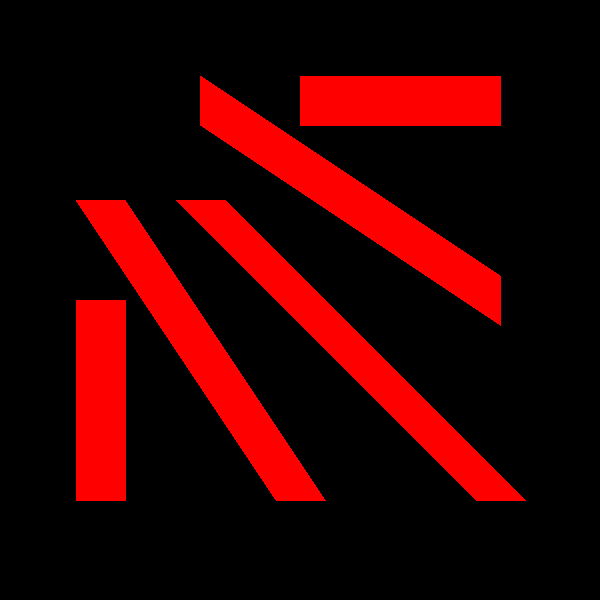
The code that generates this is attached.
This doesn't seem very useful behavior, and it make it hard to e.g. add endcaps yourself. At the very least, it's weird and the documentation doesn't lead one to expect it. Having squared-off miter joints, and the line's thickness refer to the thickness of the actual line, would be a vast improvement.
Ian
import pygame
from pygame.locals import *
pygame.init()
screen_size = [600,600]
surface = pygame.display.set_mode(screen_size)
def cappedline(surface, color, p0,p1, width=1):
pygame.draw.line(surface, color, p0,p1, width)
def get_input():
for event in pygame.event.get():
if event.type == QUIT: return False
elif event.type == KEYDOWN:
if event.key == K_ESCAPE: return False
elif event.key == K_s: pygame.image.save(surface,"test.png")
return True
def draw():
surface.fill((0,0,0))
width = 50
pygame.draw.line(surface, (255,0,0), (300,100),(500,100), width)
pygame.draw.line(surface, (255,0,0), (200,100),(500,300), width)
pygame.draw.line(surface, (255,0,0), (200,200),(500,500), width)
pygame.draw.line(surface, (255,0,0), (100,200),(300,500), width)
pygame.draw.line(surface, (255,0,0), (100,300),(100,500), width)
pygame.display.flip()
def main():
while True:
if not get_input(): break
draw()
pygame.quit()
if __name__ == "__main__": main()